Top 35 Selenium Interview Questions and Answers for Beginners 2025
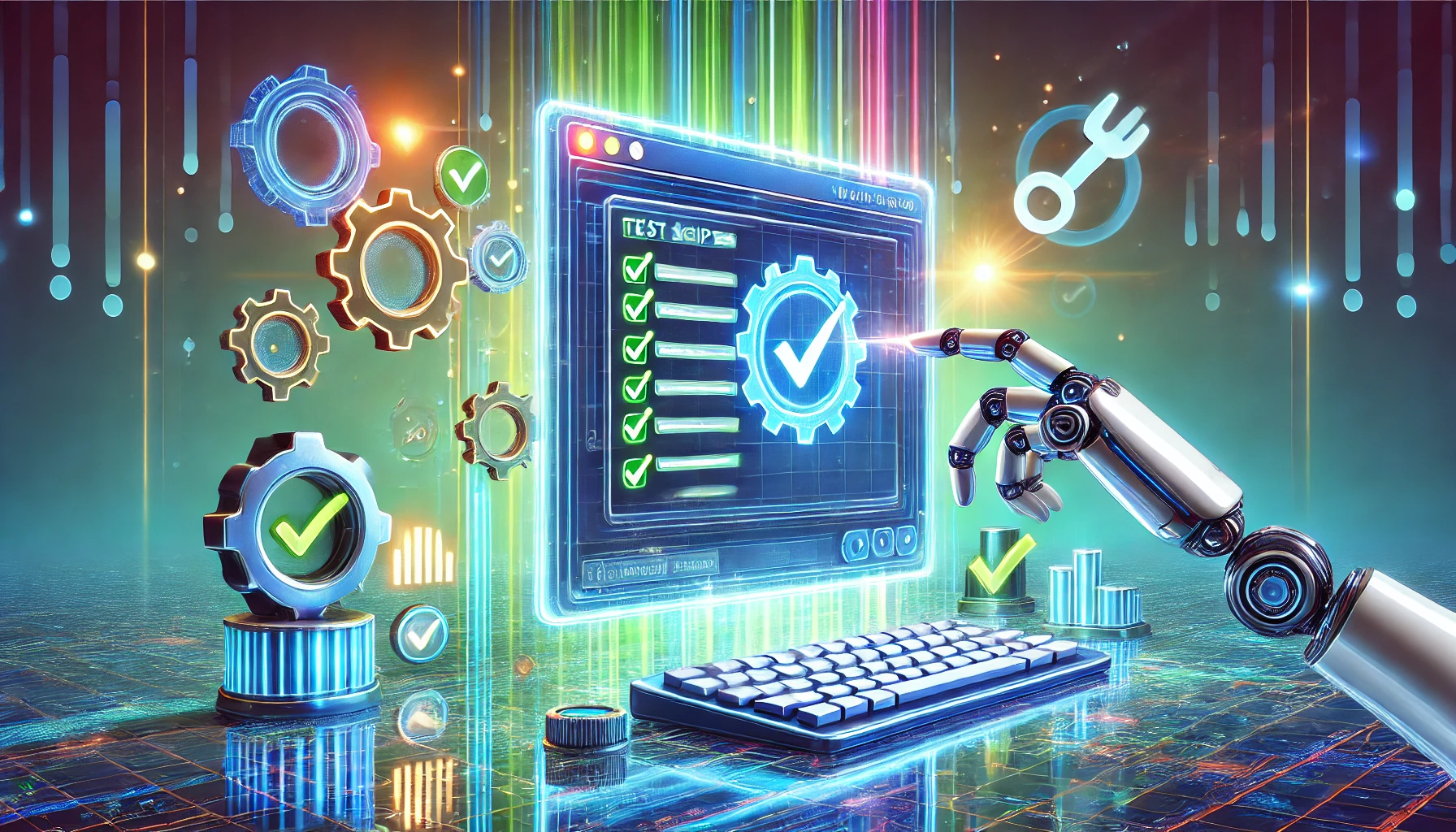
Selenium is one of the most popular tools for web automation testing. If you’re preparing for a Selenium interview, here are some beginner-friendly questions and answers to get you started:
1. What is Selenium?
Selenium is an open-source tool used for automating web applications. It supports multiple browsers like Chrome, Firefox, and Safari and can be used with various programming languages, such as Java, Python, C#, etc.
2. What are the main components of Selenium?
- Selenium IDE: A browser extension for recording and playing back tests.
- Selenium WebDriver: Used to write and run automation scripts in various programming languages.
- Selenium Grid: Enables parallel execution of tests across multiple machines and browsers.
3. What are the advantages of using Selenium?
- Open-source and free.
- Supports multiple programming languages.
- Compatible with many browsers.
- Enables integration with tools like TestNG, JUnit, and Maven.
4. What programming languages does Selenium support?
Selenium supports Java, Python, C#, Ruby, JavaScript, PHP, and more.
5. What is a WebDriver?
WebDriver is a key component of Selenium that provides an interface for interacting with web elements on a browser. It directly communicates with the browser to execute automation scripts.
6. What is the difference between Selenium IDE and Selenium WebDriver?
- Selenium IDE: Best for beginners; used for recording and playback.
- Selenium WebDriver: Requires coding; used for creating complex test scripts.
7. What is XPath in Selenium?
XPath is a way to locate elements in a web page using their path in the XML structure. It is especially useful when elements don’t have unique IDs or class names.
8. Can Selenium handle CAPTCHA?
No, Selenium cannot handle CAPTCHA as it is designed to block automated tools. However, you can integrate third-party tools or manual intervention for CAPTCHA handling.
9. How can you handle alerts and pop-ups in Selenium?
Selenium provides an Alert
interface to manage pop-ups. For example:
javaCopy codeAlert alert = driver.switchTo().alert();
alert.accept(); // To accept the alert
alert.dismiss(); // To dismiss the alert
10. What are implicit and explicit waits in Selenium?
- Implicit Wait: Tells WebDriver to wait for a certain amount of time before throwing an exception.
- Explicit Wait: Waits for a specific condition to be met before continuing.
Example of Explicit Wait:
javaCopy codeWebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("elementID")));
11. What is the difference between findElement()
and findElements()
?
- findElement(): Returns the first matching element.
- findElements(): Returns a list of all matching elements.
12. How do you handle dropdowns in Selenium?
Selenium provides the Select
class to manage dropdowns. Example:
javaCopy codeSelect dropdown = new Select(driver.findElement(By.id("dropdownID")));
dropdown.selectByVisibleText("Option 1");
13. What are some common exceptions in Selenium?
- NoSuchElementException: When the element cannot be found.
- StaleElementReferenceException: When the element is no longer attached to the DOM.
- TimeoutException: When an operation exceeds the set time.
14. How do you take a screenshot in Selenium?
Use the TakesScreenshot
interface. Example:
javaCopy codeFile screenshot = ((TakesScreenshot) driver).getScreenshotAs(OutputType.FILE);
FileUtils.copyFile(screenshot, new File("path/to/save/screenshot.png"));
15. What is Selenium Grid?
Selenium Grid allows running tests on multiple browsers and systems simultaneously, improving test efficiency.
16. What is the difference between Selenium WebDriver and Selenium RC?
- Selenium WebDriver: Directly interacts with the browser using native browser automation APIs.
- Selenium RC (Remote Control): Requires a server to interact with browsers and is now deprecated.
17. Can Selenium automate tasks on mobile applications?
Selenium alone cannot automate mobile applications. However, it can test mobile web browsers. For mobile apps, tools like Appium are used in combination with Selenium.
18. What are some limitations of Selenium?
- Cannot handle CAPTCHA or OTPs directly.
- Cannot automate desktop applications.
- No inbuilt reporting system (requires integration with tools like TestNG).
- Limited support for image-based testing.
19. How can you maximize or minimize a browser window in Selenium?
- Maximize:
javaCopy codedriver.manage().window().maximize();
- Minimize:
javaCopy codedriver.manage().window().minimize();
20. What is Page Object Model (POM) in Selenium?
POM is a design pattern where each web page is represented as a class, and its elements are defined as variables. This improves code reusability and readability.
Example:
javaCopy codepublic class LoginPage {
WebDriver driver;
By username = By.id("username");
By password = By.id("password");
By loginButton = By.id("loginBtn");
public LoginPage(WebDriver driver) {
this.driver = driver;
}
public void login(String user, String pass) {
driver.findElement(username).sendKeys(user);
driver.findElement(password).sendKeys(pass);
driver.findElement(loginButton).click();
}
}
21. What is the difference between driver.close()
and driver.quit()
?
- driver.close(): Closes the current browser window.
- driver.quit(): Closes all browser windows opened by the WebDriver and ends the session.
22. How can you switch between multiple tabs in a browser using Selenium?
Selenium uses the getWindowHandles()
method to handle multiple tabs.
Example:
javaCopy codeSet<String> windows = driver.getWindowHandles();
for (String window : windows) {
driver.switchTo().window(window);
}
23. What is Fluent Wait in Selenium?
Fluent Wait is an advanced form of Explicit Wait that allows you to define polling intervals and handle exceptions.
Example:
javaCopy codeWait<WebDriver> wait = new FluentWait<>(driver)
.withTimeout(Duration.ofSeconds(30))
.pollingEvery(Duration.ofSeconds(5))
.ignoring(NoSuchElementException.class);
WebElement element = wait.until(driver -> driver.findElement(By.id("elementID")));
24. How do you scroll down a web page in Selenium?
Use JavaScriptExecutor to perform scrolling.
Example:
javaCopy codeJavascriptExecutor js = (JavascriptExecutor) driver;
js.executeScript("window.scrollBy(0, 500);");
25. How can you upload a file using Selenium?
Use the sendKeys()
method to specify the file path to a file upload input field.
Example:
javaCopy codeWebElement uploadElement = driver.findElement(By.id("upload"));
uploadElement.sendKeys("C:\\path\\to\\file.txt");
26. How do you handle frames in Selenium?
Selenium allows switching to frames using switchTo().frame()
.
Example:
javaCopy codedriver.switchTo().frame("frameName");
// Perform actions inside the frame
driver.switchTo().defaultContent(); // Switch back to the main page
27. What are the different types of locators in Selenium?
Selenium provides these locators to identify elements:
- ID:
By.id("elementID")
- Name:
By.name("elementName")
- Class Name:
By.className("className")
- XPath:
By.xpath("//tag[@attribute='value']")
- CSS Selector:
By.cssSelector("cssSelector")
- Tag Name:
By.tagName("tagName")
- Link Text:
By.linkText("linkText")
- Partial Link Text:
By.partialLinkText("partialText")
28. How can you verify if an element is displayed, enabled, or selected in Selenium?
Use the following WebElement methods:
- isDisplayed(): Checks if the element is visible on the page.
- isEnabled(): Verifies if the element is enabled for interaction.
- isSelected(): Checks if a checkbox or radio button is selected.
29. How do you handle dynamic web elements in Selenium?
For dynamic elements where attributes like ID or name keep changing, use XPath with functions like contains()
or starts-with()
.
Example:
javaCopy codedriver.findElement(By.xpath("//button[contains(text(), 'Submit')]"));
30. What are listeners in Selenium, and why are they used?
Listeners in Selenium are used to monitor events during the test execution. For example, TestNG provides ITestListener
to log events like test start, test success, or failure.
31. How do you validate the title of a web page in Selenium?
Use the getTitle()
method.
Example:
javaCopy codeString pageTitle = driver.getTitle();
if (pageTitle.equals("Expected Title")) {
System.out.println("Title matches");
} else {
System.out.println("Title does not match");
}
32. What is the purpose of the Actions
class in Selenium?
The Actions
class is used to perform advanced user interactions like mouse hover, drag and drop, and right-click.
Example:
javaCopy codeActions actions = new Actions(driver);
actions.moveToElement(driver.findElement(By.id("hoverElement"))).perform();
33. How do you verify a tooltip in Selenium?
Retrieve the value of the title
attribute of the element.
Example:
javaCopy codeString tooltip = driver.findElement(By.id("elementID")).getAttribute("title");
System.out.println("Tooltip text: " + tooltip);
34. Can Selenium handle HTTPS websites?
Yes, Selenium can test HTTPS websites. If there are certificate errors, you can use browser-specific capabilities to bypass them (e.g., ChromeOptions or FirefoxProfile).
35. What is the use of DesiredCapabilities
in Selenium?
DesiredCapabilities
is used to configure browser properties for WebDriver. It is helpful when setting up Selenium Grid or handling special browser settings.
Example:
javaCopy codeDesiredCapabilities capabilities = new DesiredCapabilities();
capabilities.setCapability("browserName", "chrome");
Final Tips
- Always keep your code clean and readable.
- Practice writing automation scripts for different scenarios.
- Familiarize yourself with debugging techniques to fix script issues.
This guide should help beginners understand and prepare for Selenium interviews. Practice regularly, and you’ll be confident in no time!