Best Python Interview Questions for Data Analyst
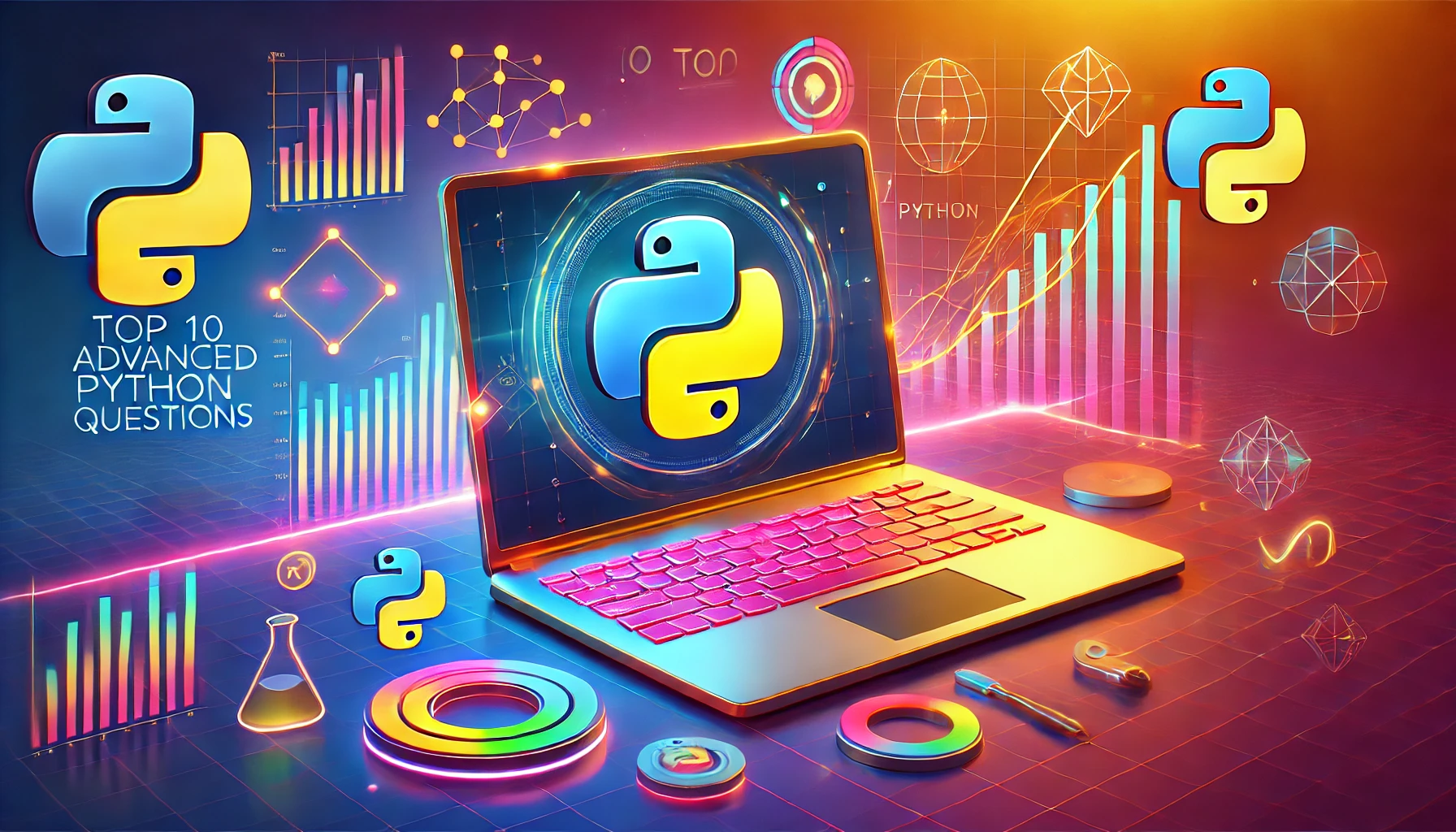
Q1. What is Python, and why is it popular in data analytics?
Python is a beginner-friendly, high-level programming language celebrated for its simplicity and readability. In data analytics, it’s a top choice because of its extensive ecosystem of libraries like pandas, NumPy, and Matplotlib, which simplify tasks such as data cleaning, analysis, and visualization. Python’s flexibility also makes it easy to integrate with databases, machine learning frameworks, and visualization tools, ensuring smooth end-to-end data workflows.
Q2. How can you install external libraries in Python?
To install libraries in Python, you use pip, Python’s package manager. For example, if you need to install the pandas library, the command is:
bashCopy codepip install pandas
To ensure compatibility, you can specify versions like pip install pandas==1.5.0
. For large projects, it’s a good idea to use virtual environments like venv
or conda
to manage dependencies cleanly.
Q3. What is pandas, and how is it used in data analysis?
Pandas is a powerful Python library designed for data manipulation and analysis. It provides two core data structures:
- Series: One-dimensional labeled arrays (similar to a column in Excel).
- DataFrame: Two-dimensional labeled data structures (similar to a spreadsheet or SQL table).
Pandas simplifies tasks like handling missing data, merging datasets, and reshaping data. For example, loading and analyzing a CSV file is effortless with pandas:
pythonCopy codeimport pandas as pd
df = pd.read_csv('data.csv')
print(df.head())
Q4. How can you read a CSV file into a DataFrame using pandas?
To read a CSV file in pandas, you use the pd.read_csv()
method. Here’s an example:
pythonCopy codeimport pandas as pd
df = pd.read_csv('file.csv')
print(df.head())
You can add parameters for customization, such as sep
for delimiters, header
to specify header rows, and usecols
to select specific columns.
Q5. What is NumPy, and why is it essential in data analysis?
NumPy (Numerical Python) is a library that provides support for fast numerical computations with multi-dimensional arrays and matrices. It’s particularly useful for performing vectorized operations, which are faster and more efficient than traditional Python loops.
For example, instead of summing a list using a loop:
pythonCopy codeimport numpy as np
arr = np.array([1, 2, 3, 4, 5])
print(arr.sum())
Q6. How do you create a NumPy array?
A NumPy array can be created using the np.array()
function. Example:
pythonCopy codeimport numpy as np
arr = np.array([10, 20, 30, 40])
print(arr)
You can also create arrays with predefined values, like zeros or random numbers:
pythonCopy codenp.zeros((3, 3)) # 3x3 matrix of zeros
np.random.rand(4) # Array of 4 random values
Q7. What is the difference between a DataFrame and a Series in pandas?
- DataFrame: A two-dimensional structure with labeled rows and columns, similar to a table in SQL or Excel.
- Series: A one-dimensional structure, similar to a single column or a list with labels.
Example:
pythonCopy codeimport pandas as pd
# Series
series = pd.Series([1, 2, 3], index=['a', 'b', 'c'])
# DataFrame
data = {'Name': ['Alice', 'Bob'], 'Age': [25, 30]}
df = pd.DataFrame(data)
Q8. How do you select specific rows and columns in pandas?
Use .iloc[]
for positional indexing or .loc[]
for label-based indexing. Example:
pythonCopy code# Select rows 2 to 4 and columns 1 to 3
subset = df.iloc[2:5, 1:3]
Q9. What is Matplotlib, and how is it used in data analysis?
Matplotlib is a versatile visualization library in Python. It allows analysts to create line plots, bar charts, histograms, scatter plots, and more.
Example of a line plot:
pythonCopy codeimport matplotlib.pyplot as plt
plt.plot([1, 2, 3], [4, 5, 6])
plt.title("Example Line Plot")
plt.show()
Q10. What is data cleaning, and why is it important?
Data cleaning involves removing or correcting inaccuracies, duplicates, and missing values in a dataset. This step ensures the data’s accuracy and reliability, enabling better decision-making and more trustworthy analysis. Tasks include:
- Removing duplicates.
- Filling missing values with mean or median.
- Standardizing data formats.
Q11. How do you check for missing values in pandas?
To identify missing values, use the isnull()
method:
pythonCopy codemissing = df.isnull().sum()
print(missing)
Q12. How do you create a histogram using Matplotlib?
Use the plt.hist()
function:
pythonCopy codeimport matplotlib.pyplot as plt
plt.hist(data, bins=10)
plt.title("Histogram Example")
plt.show()
Q13. What are some common methods for handling missing values in pandas?
In pandas, you can handle missing values in several ways:
- Remove Missing Data:
Use thedropna()
method to remove rows or columns with missing values:pythonCopy codedf_cleaned = df.dropna()
This method is suitable if missing values are few and won’t significantly impact the analysis. - Fill Missing Data:
Use thefillna()
method to replace missing values with a specific value:pythonCopy codedf_filled = df.fillna(0) # Replace with zero
- Interpolate Missing Data:
Estimate missing values using interpolation:pythonCopy codedf_interpolated = df.interpolate()
- Custom Imputation:
Fill missing values with a statistical measure like mean or median:pythonCopy codedf['column'] = df['column'].fillna(df['column'].mean())
Q14. How do you calculate descriptive statistics for a DataFrame in pandas?
Use the describe()
method to get summary statistics for numerical columns, such as mean, median, standard deviation, and percentiles.
Example:
pythonCopy codesummary_stats = df.describe()
print(summary_stats)
This method is helpful for a quick overview of the dataset’s numerical properties.
Q15. What is a histogram, and why is it useful in data analysis?
A histogram is a visual representation of the frequency distribution of numerical data. It divides data into bins (ranges) and uses bars to show the count of values in each bin.
Why is it useful?
- It reveals the distribution shape (e.g., normal, skewed).
- Identifies outliers and trends in data.
Example:
pythonCopy codeimport matplotlib.pyplot as plt
plt.hist(data, bins=10)
plt.title("Histogram Example")
plt.xlabel("Range")
plt.ylabel("Frequency")
plt.show()
Q16. What is the purpose of data visualization in data analysis?
Data visualization translates complex data into visual formats, such as charts and graphs, to:
- Communicate insights clearly to stakeholders.
- Spot patterns, trends, and anomalies.
- Make data-driven decisions faster.
Visualization tools like Matplotlib, Seaborn, and Plotly are widely used for this purpose.
Q17. How do you customize a Matplotlib plot?
You can customize Matplotlib plots by adding titles, labels, colors, and more:
pythonCopy codeimport matplotlib.pyplot as plt
plt.plot([1, 2, 3], [4, 5, 6], color='red', linestyle='--', marker='o')
plt.title("Customized Plot")
plt.xlabel("X-axis Label")
plt.ylabel("Y-axis Label")
plt.grid(True)
plt.show()
This allows you to make your plots visually appealing and informative.
Q18. What is the purpose of data normalization in data analysis?
Data normalization adjusts the scale of numerical features so they fall within a similar range, such as 0 to 1.
Why is it important?
- Prevents certain features with large values from dominating the analysis.
- Improves the performance of algorithms like k-Nearest Neighbors (k-NN) and neural networks.
Q19. How do you perform data normalization using scikit-learn?
Use MinMaxScaler
, StandardScaler
, or RobustScaler
for normalization.
Example using Min-Max Scaling:
pythonCopy codefrom sklearn.preprocessing import MinMaxScaler
scaler = MinMaxScaler()
normalized_data = scaler.fit_transform(data)
print(normalized_data)
This rescales data to a 0 to 1 range.
Q20. What is data aggregation, and why is it useful in data analysis?
Data aggregation involves grouping data based on specific criteria and calculating summary statistics for each group.
Why is it useful?
- Reduces large datasets into smaller, meaningful summaries.
- Helps identify trends and patterns in grouped data.
Example using pandas:
pythonCopy codegrouped = df.groupby('Category')['Sales'].sum()
print(grouped)
Q21. How do you filter data in a DataFrame using pandas?
You can filter rows based on conditions using boolean indexing.
Example:
pythonCopy codefiltered_data = df[df['Score'] > 90]
print(filtered_data)
This extracts rows where the ‘Score’ column has values greater than 90.
Q22. What is the purpose of data filtering in data analysis?
Data filtering allows analysts to:
- Focus on relevant subsets of data.
- Exclude irrelevant or noisy information.
- Simplify analysis and visualization by reducing dataset size.
TOP 10 advanced Python interview questions for data analysts
Q1. How would you optimize a large dataset in pandas to improve performance?
When working with large datasets in pandas, performance optimization is crucial. Here are some tips:
- Use Efficient Data Types: Convert columns to appropriate data types. For example:pythonCopy code
df['column'] = df['column'].astype('category') # Converts to categorical data type
- Load Data in Chunks: Use the
chunksize
parameter inpd.read_csv()
to process large files in smaller portions:pythonCopy codefor chunk in pd.read_csv('large_file.csv', chunksize=10000): process(chunk)
- Vectorized Operations: Avoid loops and use built-in functions for computations.pythonCopy code
df['new_column'] = df['col1'] + df['col2']
- Use Dask or PySpark: For very large datasets, consider tools like Dask or PySpark for distributed computing.
Q2. Explain the concept of MultiIndex in pandas. When would you use it?
A MultiIndex is a pandas object that allows hierarchical indexing. It’s useful when working with data that has multiple levels of categorization.
Example Use Case:
If you’re analyzing sales data across regions and years, MultiIndex allows you to organize and analyze the data more effectively.
pythonCopy codeimport pandas as pd
data = {
'Region': ['North', 'North', 'South', 'South'],
'Year': [2020, 2021, 2020, 2021],
'Sales': [200, 250, 300, 350]
}
df = pd.DataFrame(data)
df.set_index(['Region', 'Year'], inplace=True)
print(df)
Advantages:
- Simplifies working with multi-level data.
- Enables advanced slicing and filtering operations.
Q3. What are lambda functions in Python, and how are they used in data analysis?
Lambda functions are anonymous functions defined using the lambda
keyword. They are commonly used for short, one-line operations.
Example:
Using a lambda function to create a new column in pandas:
pythonCopy codedf['adjusted_sales'] = df['Sales'].apply(lambda x: x * 1.1)
Use Cases in Data Analysis:
- Applying custom calculations to DataFrame columns.
- Filtering data based on conditions.
- Using in combination with functions like
map()
orfilter()
.
Q4. How do you handle outliers in a dataset?
Outliers can distort data analysis, so it’s important to handle them appropriately:
- Identify Outliers:
- Using statistical methods like z-scores or the IQR method:pythonCopy code
Q1 = df['column'].quantile(0.25) Q3 = df['column'].quantile(0.75) IQR = Q3 - Q1 outliers = df[(df['column'] < Q1 - 1.5 * IQR) | (df['column'] > Q3 + 1.5 * IQR)]
- Using statistical methods like z-scores or the IQR method:pythonCopy code
- Handle Outliers:
- Remove: Drop rows containing outliers.
- Cap/Impute: Replace outliers with the mean, median, or a capped value.
df['column'] = df['column'].clip(lower=Q1 - 1.5 * IQR, upper=Q3 + 1.5 * IQR)
Q5. How can you merge or join two datasets in pandas? What are the different types of joins?
To merge two datasets, use the pd.merge()
function in pandas. The types of joins are:
- Inner Join: Includes only matching rows from both datasets.
- Left Join: Includes all rows from the left dataset and matching rows from the right.
- Right Join: Includes all rows from the right dataset and matching rows from the left.
- Outer Join: Includes all rows from both datasets.
Example:
pythonCopy codedf1 = pd.DataFrame({'ID': [1, 2], 'Name': ['Alice', 'Bob']})
df2 = pd.DataFrame({'ID': [1, 3], 'Score': [90, 95]})
result = pd.merge(df1, df2, on='ID', how='inner')
print(result)
Q6. What is the difference between apply()
, map()
, and applymap()
in pandas?
apply()
: Applies a function along an axis (rows or columns) of a DataFrame.pythonCopy codedf['adjusted'] = df['Sales'].apply(lambda x: x * 1.1)
map()
: Used with Series to apply functions or map values.pythonCopy codedf['Category'] = df['Region'].map({'North': 'A', 'South': 'B'})
applymap()
: Applies a function element-wise to all DataFrame cells.pythonCopy codedf = df.applymap(lambda x: x * 2 if isinstance(x, int) else x)
Q7. How do you handle categorical variables in data analysis?
Categorical variables need to be converted into numerical formats for analysis or modeling.
- Label Encoding: Assigns integers to each category.pythonCopy code
from sklearn.preprocessing import LabelEncoder le = LabelEncoder() df['Category'] = le.fit_transform(df['Category'])
- One-Hot Encoding: Converts categories into binary columns.pythonCopy code
df = pd.get_dummies(df, columns=['Category'])
Q8. What are generators in Python, and how can they be useful in data analysis?
Generators are special functions that yield items one at a time using the yield
keyword.
Advantages in Data Analysis:
- Memory-efficient for processing large datasets.
- Can be used to create custom data pipelines.
Example:
pythonCopy codedef square_numbers(nums):
for num in nums:
yield num ** 2
squares = square_numbers([1, 2, 3])
print(list(squares))
Q9. Explain the use of SQL queries in pandas using pandasql
.
The pandasql
library allows you to write SQL queries directly on pandas DataFrames.
Example:
pythonCopy codeimport pandasql as ps
query = "SELECT * FROM df WHERE Sales > 200"
result = ps.sqldf(query, locals())
print(result)
This is helpful when you’re comfortable with SQL syntax or want to perform complex filtering and aggregations.
Q10. How can you schedule and automate data analysis tasks in Python?
Use the schedule
library or cron jobs to automate tasks.
Example:
pythonCopy codeimport schedule
import time
def job():
print("Task running...")
schedule.every().day.at("10:00").do(job)
while True:
schedule.run_pending()
time.sleep(1)
Automation ensures tasks like daily report generation or data updates are performed consistently without manual intervention.
These advanced questions are designed to test deeper knowledge of Python for data analysis while keeping explanations straightforward and examples relevant to real-world scenarios.